For this use case the external process needs to get product price from Merchandizing system running on AS/400.
Mule demo code
-
Create Mule application that:
-
listens for HTTP requests with HTTP URL parameter productCode
http://localhost:8081/getProductPrice?productCode=CHAIR
-
sends request to AS/400 data queue
-
waits for response from AS/400 in the response data queue
-
returns the product price back to HTTP response
Status=OK;100.00
-
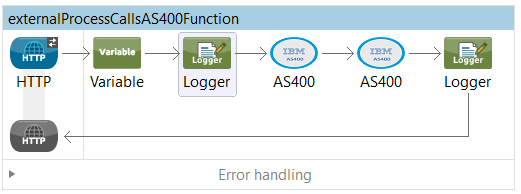
<?xml version="1.0" encoding="UTF-8"?>
<mule xmlns:json="http://www.mulesoft.org/schema/mule/json"
xmlns:tracking="http://www.mulesoft.org/schema/mule/ee/tracking"
xmlns:metadata="http://www.mulesoft.org/schema/mule/metadata"
xmlns:data-mapper="http://www.mulesoft.org/schema/mule/ee/data-mapper"
xmlns:ws="http://www.mulesoft.org/schema/mule/ws" xmlns:dw="http://www.mulesoft.org/schema/mule/ee/dw"
xmlns:http="http://www.mulesoft.org/schema/mule/http" xmlns:as400="http://www.mulesoft.org/schema/mule/as400"
xmlns="http://www.mulesoft.org/schema/mule/core" xmlns:doc="http://www.mulesoft.org/schema/mule/documentation"
xmlns:spring="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.mulesoft.org/schema/mule/json http://www.mulesoft.org/schema/mule/json/current/mule-json.xsd
http://www.mulesoft.org/schema/mule/ws http://www.mulesoft.org/schema/mule/ws/current/mule-ws.xsd
http://www.mulesoft.org/schema/mule/ee/dw http://www.mulesoft.org/schema/mule/ee/dw/current/dw.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-current.xsd
http://www.mulesoft.org/schema/mule/as400 http://www.mulesoft.org/schema/mule/as400/current/mule-as400.xsd
http://www.mulesoft.org/schema/mule/core http://www.mulesoft.org/schema/mule/core/current/mule.xsd
http://www.mulesoft.org/schema/mule/http http://www.mulesoft.org/schema/mule/http/current/mule-http.xsd
http://www.mulesoft.org/schema/mule/ee/data-mapper http://www.mulesoft.org/schema/mule/ee/data-mapper/current/mule-data-mapper.xsd
http://www.mulesoft.org/schema/mule/ee/tracking http://www.mulesoft.org/schema/mule/ee/tracking/current/mule-tracking-ee.xsd" version="EE-3.7.0">
<as400:config name="AS/400__Configuration_type_strategy"
endpoint="${endpoint}" userid="${userid}" password="${password}"
doc:name="AS/400: Configuration type strategy" libraryList="${libl}">
<reconnect-forever />
</as400:config>
<http:listener-config name="HTTP_Listener_Configuration"
host="0.0.0.0" port="8081" doc:name="HTTP Listener Configuration" />
<ws:consumer-config name="Web_Service_Consumer"
wsdlLocation="http://www.webservicex.net/CurrencyConvertor.asmx?wsdl"
service="CurrencyConvertor" port="CurrencyConvertorSoap"
serviceAddress="http://www.webservicex.net/CurrencyConvertor.asmx"
doc:name="Web Service Consumer" />
<flow name="externalProcessCallsAS/400Function">
<http:listener config-ref="HTTP_Listener_Configuration"
path="/getProductPrice" doc:name="HTTP" />
<set-variable variableName="key"
value="#[java.util.UUID.randomUUID().toString()]" doc:name="Variable" />
<logger
message="Product code #[message.inboundProperties.'http.query.params'.productCode] Key #[flowVars['key']]"
level="INFO" doc:name="Logger" />
<as400:write-data-queue config-ref="AS/400__Configuration_type_strategy"
dtaq="${req.dtaq}" library="${library}"
dqEntry="#[message.inboundProperties.'http.query.params'.productCode]"
doc:name="AS/400" dtaqKey="#[flowVars['key']]" />
<as400:read-data-queue-processor
config-ref="AS/400__Configuration_type_strategy" doc:name="AS/400"
dtaq="${res.dtaq}" dtaqKey="#[flowVars['key']]" dtaqKeySearchType="EQUAL"
dtaqwaittime="15" library="${library}" />
<logger message="Response #[payload]" level="INFO" doc:name="Logger" />
</flow>
</mule>
AS/400 demo code
-
Create a new table that will hold product prices
create table DEMO02P ( productCd char(30) not null primary key, prodPrc dec(11, 2) not null);
-
Add test products, for example CHAIR, TABLE, TV
-
Create an AS/400 program that
-
listens for new messages in request data queue
-
looks up product price based on product code sent in request body
-
returns status and price to the response data queue using the same key as request data queue
-
H DEBUG DATFMT(*ISO) bnddir('QC2LE')
fdemo02p if e k disk rename(demo02p:priceRec)
d sndDtaQ pr ExtPgm( 'QSNDDTAQ' )
d DQName 10 Const
d DQLibl 10 Const
d DQLength 5 0 Const
d DQData 255 Const
d DQKeyLength 3p 0 options(*nopass) const
d DQKey 20 options(*nopass) const
d rcvDtaQ pr ExtPgm('QRCVDTAQ')
d Dtaqnam 10a const
d Dtaqlib 10a const
d Dtaqlen 5p 0
d Data 255
d WaitTime 5p 0 const
d Keyorder 2a options(*nopass) const
d Keylen 3p 0 options(*nopass) const
d Key 20 options(*nopass) const
d Senderlen 3p 0 options(*nopass) const
d Sender 1 options(*nopass) const
* constants for this sample, replace with soft-coded parms
d c#reqDtaQ s 10 inz('DEMO02REQ')
d c#reqDtaQLib s 10 inz('MULE400DEV')
d c#resDtaQ s 10 inz('DEMO02RES')
d c#resDtaQLib s 10 inz('MULE400DEV')
d c#waitsec s 5p 0 inz(15)
d msgOut s 255
d msgIn s 255
d msgKey s 20
d msgInLen s 5p 0
/free
dow msgIn <> 'STOP';
// get message from DQ
msgKey = *blanks;
msgIn = *blanks;
rcvdtaq (c#reqDtaQ: c#reqDtaQLib: msgInLen : msgIn : c#waitsec :
'GE': %size(msgKey) : msgKey : 0 : ' ');
// Request for new product price
if msgInLen > 0 and %trim(msgIn) <> 'STOP';
chain (%trim(msgIn)) priceRec;
if %found;
msgOut = 'Status=OK;' + %char(prodPrc);
else;
msgOut = 'Status=FAILED;Product ' + %trim(msgIn)
+ ' not found';
endif;
snddtaq (c#resDtaQ : c#resDtaQLib : %size(msgOut) : msgOut :
%size(msgKey ) : msgKey );
endif; // msgInLen > 0
enddo; // *in03 = off
*inlr = *on;
return;
/end-free
-
Run demo:
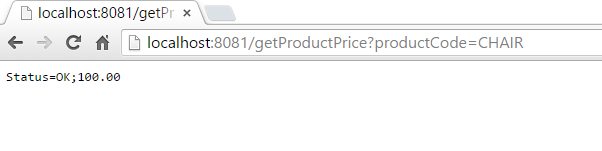